Automating Marketing Emails with Next.js
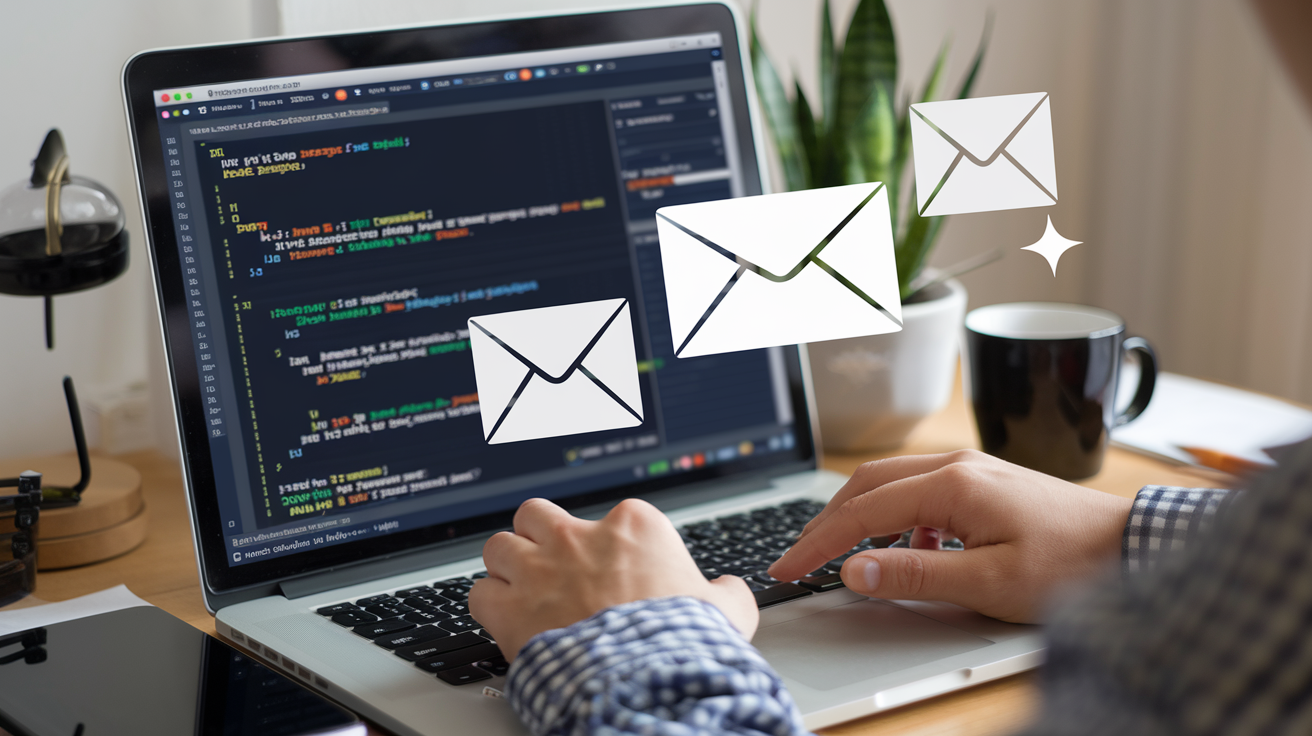
In the rapidly evolving landscape of web development, automating marketing emails has become a crucial component for enhancing user engagement and driving business growth. Leveraging the capabilities of Next.js, a powerful React framework, developers can seamlessly integrate email automation into their applications, providing a robust solution for managing communication workflows.
Next.js offers a versatile environment for building server-rendered React applications, making it an ideal choice for implementing email functionalities. By utilizing various email service providers (ESPs) such as SendGrid, Mailgun, and Mailchimp, developers can offload the complexities of email delivery and focus on crafting personalized and targeted email campaigns. These platforms provide comprehensive APIs and tools that facilitate the sending of transactional and marketing emails at scale, ensuring reliable delivery and real-time analytics.
The integration of email automation in Next.js applications is further simplified by leveraging popular Node.js libraries like Nodemailer. Nodemailer offers a straightforward approach to configuring SMTP servers and sending emails, making it a preferred choice for developers seeking a customizable and cost-effective solution. Additionally, services like EmailJS enable client-side email sending, allowing for dynamic and interactive email functionalities without the need for server-side code.
By automating marketing emails, businesses can enhance user experience through timely notifications, personalized content, and efficient communication strategies. This automation not only streamlines marketing efforts but also provides valuable insights into user interactions, enabling data-driven decision-making.
In this report, we will explore the various methodologies and best practices for automating marketing emails using Next.js. We will delve into the technical aspects of integrating different ESPs, configuring email-sending libraries, and implementing scalable email workflows. Through practical examples and code snippets, this report aims to equip developers and technical decision-makers with the knowledge required to harness the full potential of email automation in their Next.js applications.
You can also visit Oncely.com to find more Top Trending AI Tools. Oncely partners with software developers and companies to present exclusive deals on their products. One unique aspect of Oncely is its “Lifetime Access” feature, where customers can purchase a product once and gain ongoing access to it without any recurring fees. Oncely also provides a 60-day money-back guarantee on most purchases, allowing customers to try out the products and services risk-free.
Oncely are hunting for the most fantastic AI & Software lifetime deals like the ones below or their alternatives:

Table of Contents
- Setting Up Next.js Project for Email Automation
- Installing Node.js and Creating a Next.js Project
- Exploring the Project Structure
- Configuring Environment Variables
- Integrating Email Service Providers
- Using Mailgun
- Using Nodemailer
- Implementing Email Automation Logic
- Setting Up Triggers
- Designing Email Templates
- Testing and Deployment
- Integrating Email Service Providers with Next.js
- Choosing the Right Email Service Provider
- SendGrid
- Mailgun
- Nodemailer
- EmailJS
- Implementing Email Automation with Next.js
- Setting Up API Routes
- Designing Email Templates
- Scheduling Email Campaigns
- Monitoring and Optimizing Email Campaigns
- Enhancing Deliverability and SEO
- Choosing the Right Email Service Provider
- Creating and Testing Email Sending Functionality
- Developing Email Sending Functionality
- Setting Up Next.js API Routes
- Implementing Email Sending Logic
- Testing Email Sending Functionality
- Unit Testing Email Logic
- Integration Testing with Email Sandboxes
- Ensuring Reliable Email Delivery
- Configuring Email Service Providers
- Implementing Deliverability Best Practices
- Monitoring and Optimizing Email Campaigns
- Using Analytics and Reporting Tools
- A/B Testing and Iterative Improvements
- Security and Compliance Considerations
- Protecting Sensitive Information
- Adhering to Legal Regulations
- Developing Email Sending Functionality
Setting Up Next.js Project for Email Automation
Installing Node.js and Creating a Next.js Project
To begin automating marketing emails with Next.js, the first step is to set up a Next.js project. This requires Node.js, a JavaScript runtime built on Chrome's V8 JavaScript engine, which is essential for running Next.js applications. Ensure that Node.js is installed on your system by downloading the latest LTS version from the official Node.js website. Once Node.js is installed, you can create a new Next.js project by running the following command in your terminal:
npx create-next-app@latest my-email-automation-app
This command initializes a new Next.js project with the default project structure and installs the necessary dependencies. The project structure includes essential folders and files such as pages
, public
, and styles
, which are crucial for building a Next.js application.
Exploring the Project Structure
After creating the Next.js project, navigate into the project directory using:
cd my-email-automation-app
Inside the project directory, you will find several important files and folders:
pages/
: Contains the application's pages. Each file in this directory represents a route in the application.public/
: Used for static assets like images and fonts.styles/
: Contains global styles and CSS modules.
Understanding this structure is vital for organizing your email automation logic effectively. For instance, you might place email-related API routes in the pages/api
directory, which is specifically designed for serverless functions in Next.js.
Configuring Environment Variables
Email automation often requires sensitive information such as API keys and SMTP credentials. To manage these securely, Next.js supports environment variables. Create a .env.local
file in the root of your project to store these variables:
MAILGUN_API_KEY=your-mailgun-api-key
MAILGUN_DOMAIN=your-mailgun-domain
Ensure that this file is added to your .gitignore
to prevent sensitive information from being exposed in your version control system. Using environment variables allows you to access these credentials in your application code without hardcoding them, enhancing security and flexibility.
Integrating Email Service Providers
Next.js can be integrated with various email service providers to automate marketing emails. Popular choices include Mailgun, Mailchimp, and Nodemailer. Each provider offers unique features and integration methods.
Using Mailgun
Mailgun is a powerful email API service that simplifies sending, receiving, and tracking emails. To integrate Mailgun with your Next.js project, install the mailgun-js
package:
npm install mailgun-js
In your API route, you can set up Mailgun to send emails:
import mailgun from 'mailgun-js';
const mg = mailgun({ apiKey: process.env.MAILGUN_API_KEY, domain: process.env.MAILGUN_DOMAIN });
export default async function handler(req, res) {
const data = {
from: 'Excited User <[email protected]>',
to: '[email protected], YOU@YOUR_DOMAIN_NAME',
subject: 'Hello',
text: 'Testing some Mailgun awesomeness!'
};
mg.messages().send(data, function (error, body) {
if (error) {
res.status(500).json({ error: error.message });
} else {
res.status(200).json({ message: 'Email sent successfully', body });
}
});
}
This setup allows you to send emails programmatically from your Next.js application, leveraging Mailgun's robust infrastructure.
Using Nodemailer
Nodemailer is another popular choice for sending emails in Node.js applications. It supports various transport methods, including SMTP and Gmail. To use Nodemailer, install it in your project:
npm install nodemailer
Configure Nodemailer in a separate module, such as lib/send-mail.js
:
import nodemailer from 'nodemailer';
const transporter = nodemailer.createTransport({
service: 'gmail',
auth: {
user: process.env.EMAIL_USER,
pass: process.env.EMAIL_PASS
}
});
export const sendEmail = async (options) => {
const mailOptions = {
from: 'Your Name <[email protected]>',
to: options.to,
subject: options.subject,
text: options.text
};
await transporter.sendMail(mailOptions);
};
This configuration allows you to send emails using Gmail's SMTP service. Ensure that you have enabled "Less secure app access" in your Google account settings or use an app-specific password if two-factor authentication is enabled.
Implementing Email Automation Logic
With the email service provider integrated, the next step is to implement the logic for automating marketing emails. This involves setting up triggers and workflows that determine when and how emails are sent.
Setting Up Triggers
Triggers can be based on various user actions or events, such as signing up for a newsletter, completing a purchase, or reaching a specific milestone. In Next.js, you can use API routes to handle these events and initiate email sending.
For example, to send a welcome email when a user signs up, you might create an API route like pages/api/signup.js
:
import { sendEmail } from '../../lib/send-mail';
export default async function handler(req, res) {
if (req.method === 'POST') {
const { email, name } = req.body;
try {
await sendEmail({
to: email,
subject: 'Welcome to Our Service!',
text: `Hi ${name}, welcome to our service! We're glad to have you on board.`
});
res.status(200).json({ message: 'Welcome email sent successfully' });
} catch (error) {
res.status(500).json({ error: error.message });
}
} else {
res.setHeader('Allow', ['POST']);
res.status(405).end(`Method ${req.method} Not Allowed`);
}
}
This route listens for POST requests, extracts user data from the request body, and sends a welcome email using the sendEmail
function.
Designing Email Templates
Effective email automation requires well-designed email templates. These templates should be personalized and engaging to maximize user interaction. You can use HTML and CSS to design your templates, and many email service providers offer template editors to simplify this process.
For instance, Mailgun allows you to create and manage templates directly in their dashboard, which can then be referenced in your API calls.
Testing and Deployment
Before deploying your Next.js application, thoroughly test the email automation functionality to ensure reliability and accuracy. Use test accounts and scenarios to simulate real-world usage and verify that emails are sent as expected.
Once testing is complete, deploy your application using a platform like Vercel, which offers seamless integration with Next.js. Ensure that all environment variables are correctly configured in the deployment environment to maintain functionality.
By following these steps, you can effectively set up a Next.js project for email automation, enabling you to automate marketing emails and enhance user engagement. This setup leverages the power of Next.js and modern email service providers to deliver scalable and efficient email solutions.
Integrating Email Service Providers with Next.js
Choosing the Right Email Service Provider
When integrating email service providers with Next.js, selecting the right provider is crucial for ensuring reliable and efficient email delivery. Several providers offer robust APIs and features that cater to different needs. Among the most popular are SendGrid, Mailgun, Mailgun, Nodemailer, and EmailJS. Each of these services provides unique features that can be leveraged to automate marketing emails effectively.
SendGrid
SendGrid is renowned for its powerful API and comprehensive email infrastructure, making it a preferred choice for many developers. It supports transactional and marketing emails, offering features like dynamic templates and scheduling. SendGrid's API can be easily integrated with Next.js serverless functions, allowing developers to send high-volume emails without managing infrastructure. The service also provides robust analytics and event tracking capabilities, which can be integrated into Next.js applications to monitor email performance and optimize campaigns (SendGrid NextJS Guide).
Mailgun
Mailgun offers a simple API for sending, receiving, and tracking emails, making it an excellent choice for developers looking to incorporate seamless email functionalities into their applications. It supports transactional notifications, marketing workflows, and scalable support systems. Mailgun's integration with Next.js allows developers to process inbound webhooks, enabling real-time automation based on email events such as opens, clicks, and bounces (Mailgun and NextJS).
Nodemailer
Nodemailer is a popular Node.js library known for its flexibility and ease of use. It is particularly useful for sending emails via SMTP, making it a suitable choice for developers who prefer to manage their own email infrastructure. Nodemailer can be integrated with Next.js to send emails through various SMTP services, including Gmail. This integration allows developers to automate emails like account verification and password recovery, enhancing user experience and engagement (Nodemailer Integration).
EmailJS
EmailJS allows developers to send emails directly from JavaScript without needing a backend server. This makes it ideal for client-side applications where server infrastructure is not available. EmailJS connects to popular email services like Gmail and Outlook, simplifying the process of sending transactional emails directly from the frontend. It is particularly useful for contact forms and feedback forms in Next.js applications (EmailJS Integration).
Implementing Email Automation with Next.js
Once the appropriate email service provider is selected, the next step is to implement email automation within a Next.js application. This involves setting up API routes, designing email templates, and scheduling email campaigns.
Setting Up API Routes
Next.js provides built-in API routes that can be used to handle backend logic for email automation. These routes can be configured to trigger emails based on user actions, such as signing up or making a purchase. For example, an API route can be set up to send a welcome email when a new user registers. This is achieved by connecting the API route to the signup form and using the email service provider's API to send the email (Next.js API Routes).
Designing Email Templates
Creating custom email templates is essential for maintaining consistency in branding and design across different campaigns. With Next.js, developers can use React components to design responsive and customizable email templates. These templates can be dynamically updated to personalize content for individual recipients based on their preferences or actions. Tools like React Email or MJML can be used to create these templates, which can then be integrated into email automation workflows (Custom Email Templates).
Scheduling Email Campaigns
Scheduling email campaigns is crucial for engaging the audience at the right moments. Next.js can be used alongside task schedulers like node-cron to automate the sending of emails at specific times. This allows developers to plan campaigns in advance and ensure timely delivery of marketing emails. The node-cron package can be installed and configured to schedule tasks that trigger email sending at predetermined intervals (Scheduling with node-cron).
Monitoring and Optimizing Email Campaigns
Monitoring the performance of email campaigns is essential for optimizing their effectiveness. Key metrics such as open rates, click-through rates (CTR), and conversion rates should be tracked to gather insights and make data-driven decisions. Email service providers like SendGrid and Mailgun offer analytics tools that can be integrated into Next.js applications to monitor these metrics. By analyzing the data, developers can identify areas for improvement and optimize campaigns for better performance (Monitoring Campaigns).
Enhancing Deliverability and SEO
Improving email deliverability and optimizing landing pages for SEO are critical components of a successful email marketing strategy. Next.js's server-side rendering (SSR) capability ensures that email content is delivered faster, reducing the chances of being flagged as spam. This improves the overall deliverability of emails, leading to better engagement rates. Additionally, Next.js enables the creation of SEO-optimized landing pages that load quickly and rank higher in search engine results, boosting conversion rates from email campaigns (SEO and Deliverability).
By integrating email service providers with Next.js, developers can automate marketing emails efficiently, ensuring timely delivery, personalized content, and improved engagement with their audience. The combination of Next.js's capabilities and the features offered by email service providers creates a powerful framework for executing successful email marketing campaigns.
Creating and Testing Email Sending Functionality
Developing Email Sending Functionality
To automate marketing emails with Next.js, it is essential to develop a robust email sending functionality. This involves setting up serverless functions using Next.js API routes, which allow secure interaction with email service providers like SendGrid, Mailgun, or AWS SES. These API routes act as backend endpoints that handle email sending logic, ensuring that sensitive information such as API keys remains secure and not exposed to the client-side.
Setting Up Next.js API Routes
Next.js API routes are a powerful feature that enables the creation of serverless functions. These routes are defined within the pages/api
directory and can be accessed via HTTP requests. For instance, to send emails using SendGrid, you would create a file such as pages/api/send-email.js
and implement the email sending logic within it. This setup allows you to securely use the SendGrid API key by keeping it on the server side, thus preventing unauthorized access (Space Jelly).
Implementing Email Sending Logic
The email sending logic typically involves initializing the email service provider's SDK, setting up the email parameters (such as sender, recipient, subject, and body), and invoking the send method. For example, using SendGrid, you would initialize the SDK with sgMail.setApiKey(process.env.SENDGRID_API_KEY)
and then use sgMail.send()
to dispatch the email (Upmostly).
Testing Email Sending Functionality
Testing is a critical phase in developing email automation to ensure that emails are sent correctly and reliably. It involves both unit testing of the email sending logic and integration testing to verify the end-to-end functionality.
Unit Testing Email Logic
Unit testing focuses on verifying the correctness of the email sending logic in isolation. This can be achieved using testing frameworks like Jest or Mocha. Mocking libraries such as nock
can simulate API responses from the email service provider, allowing you to test how your application handles different scenarios, such as successful email delivery or API errors (Mailsac).
Integration Testing with Email Sandboxes
Integration testing involves testing the complete email sending process, including the interaction with the email service provider. Email sandbox services like Mailsac or Mailtrap provide a safe environment to capture and inspect emails sent during testing. These services prevent accidental email delivery to real users and allow developers to verify email content, headers, and other metadata (Mailsac).
Ensuring Reliable Email Delivery
Reliable email delivery is crucial for effective marketing automation. This involves configuring the email service provider correctly, implementing best practices for deliverability, and monitoring email performance.
Configuring Email Service Providers
Proper configuration of the email service provider is essential to ensure emails are delivered to the recipient's inbox rather than being marked as spam. This includes setting up SPF, DKIM, and DMARC records for your domain, which authenticate your emails and improve deliverability (NextJS Starter).
Implementing Deliverability Best Practices
To enhance email deliverability, it is important to follow best practices such as maintaining a clean email list, avoiding spammy content, and personalizing emails. Additionally, using double opt-in for subscriptions and providing easy unsubscribe options can help maintain a positive sender reputation (NextJS Starter).
Monitoring and Optimizing Email Campaigns
Monitoring email campaigns is vital to understand their effectiveness and make data-driven improvements. This involves tracking key metrics such as open rates, click-through rates, and bounce rates.
Using Analytics and Reporting Tools
Most email service providers offer analytics and reporting tools that provide insights into email performance. These tools can help identify trends, such as which subject lines or content types result in higher engagement, allowing you to optimize future campaigns (NextJS Starter).
A/B Testing and Iterative Improvements
A/B testing is a powerful technique to optimize email campaigns by comparing different versions of an email to see which performs better. By testing variables such as subject lines, call-to-action buttons, or email layouts, you can make iterative improvements to enhance engagement and conversion rates (Stackademic).
Security and Compliance Considerations
Ensuring the security and compliance of your email automation system is crucial to protect user data and adhere to regulations such as GDPR or CAN-SPAM.
Protecting Sensitive Information
Sensitive information, such as API keys and user data, should be stored securely using environment variables and encrypted storage solutions. Additionally, implementing HTTPS for all API requests ensures data is transmitted securely (Space Jelly).
Adhering to Legal Regulations
Compliance with legal regulations involves obtaining user consent for email communications, providing clear unsubscribe options, and ensuring that all marketing emails include the necessary legal information, such as the sender's physical address (SuprSend).
By following these guidelines, developers can create and test robust email sending functionality in Next.js applications, ensuring effective and compliant marketing email automation.
References
- https://blog.mailsac.com/guide-to-stress-free-email-testing-with-next-js/
- https://mydevpa.ge/blog/how-to-send-emails-using-next-14-resend-and-react-email
- https://blog.stackademic.com/automate-200-emails-daily-nodemailer-next-js-13-integration-c7773ab63d5d
- https://nextjsstarter.com/blog/mailgun-npm-and-nextjs-a-perfect-pair-for-email-automation/
- https://www.learnwithjason.dev/serverless-marketing-automation-for-react
- https://www.suprsend.com/post/how-to-send-transactional-emails-with-mailchimp-in-next-js-with-code-examples
- https://spacejelly.dev/posts/how-to-send-emails-with-sendgrid-and-next-js-serverless-functions-for-a-contact-form
- https://nextjsstarter.com/blog/sendgrid-nextjs-guide-building-robust-email-functions/
- https://www.suprsend.com/post/how-to-implement-email-sending-in-next-js-with-aws-ses
- https://upmostly.com/next-js/email-made-easy-a-step-by-step-guide-to-sending-emails-from-next-js
- https://thedeveloperblog.vercel.app/post/how-to-send-emails-in-a-next-js-13-app-using-email-js
- https://blog.logrocket.com/testing-next-js-apps-jest/
- https://medium.com/@gizemcandemir3/mastering-next-js-unit-testing-a-comprehensive-guide-a52b6927e105