How to Redirect in PHP: What You Need to Know
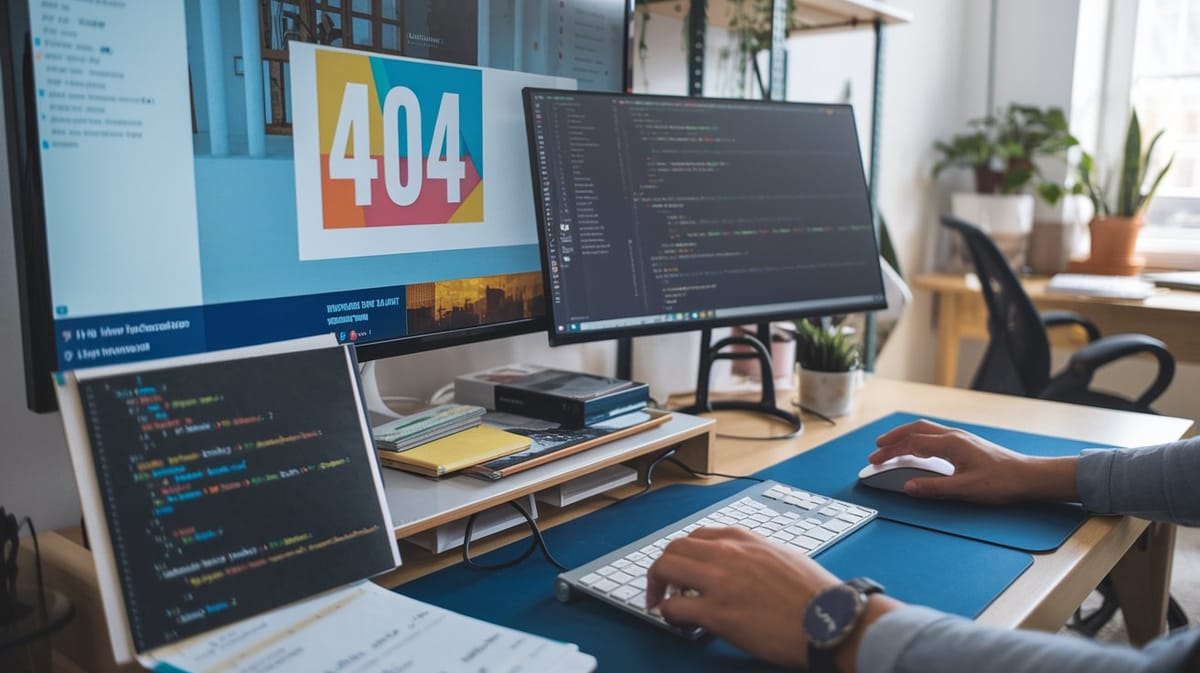
Redirecting users from one URL to another is a common and essential task in web development. PHP, as a versatile server-side scripting language, provides developers with powerful tools to implement redirects efficiently. Whether you are moving content, restructuring your website, or optimizing for SEO, understanding how to create and manage redirects in PHP is critical. This report delves into the technical aspects, best practices, and potential pitfalls of PHP redirects, providing a comprehensive guide to ensure smooth and effective implementation.
You can also visit Oncely.com to find more Top Trending AI Tools. Oncely partners with software developers and companies to present exclusive deals on their products. One unique aspect of Oncely is its “Lifetime Access” feature, where customers can purchase a product once and gain ongoing access to it without any recurring fees. Oncely also provides a 60-day money-back guarantee on most purchases, allowing customers to try out the products and services risk-free.
Oncely is hunting for the most fantastic AI & Software lifetime deals like the ones below or their alternatives:

What is a PHP Redirect?
A PHP redirect is a mechanism that allows developers to instruct a user's browser to navigate to a different URL. This is achieved by modifying the HTTP response headers sent from the server to the client. Redirects are commonly used in scenarios such as:
- Moved or Deleted Content: Redirect users to an alternative page when a URL is no longer valid.
- SEO Optimization: Preserve search engine rankings by signaling permanent or temporary changes in URL structure.
- User Experience: Guide users to the correct content, such as language-specific pages or device-optimized versions.
PHP redirects are implemented using the header()
function, which sends raw HTTP headers to the browser. This function is versatile and can be used to specify the type of redirect (e.g., permanent or temporary) and the destination URL.
Types of Redirects
Understanding the different types of redirects is crucial for selecting the appropriate one for your use case. The two most commonly used HTTP status codes for redirects are:
1. 301 Redirect (Permanent Redirect)
A 301 redirect signals that a URL has been permanently moved to a new location. This type of redirect is ideal for scenarios such as:
- Permanently changing the URL of a webpage.
- Migrating to a new domain.
- Switching from HTTP to HTTPS.
- Resolving duplicate content issues (e.g., non-www to www URLs).
From an SEO perspective, a 301 redirect helps preserve the "link equity" or ranking power of the original URL.
2. 302 Redirect (Temporary Redirect)
A 302 redirect indicates that the URL change is temporary. Use cases include:
- Redirecting users during website maintenance.
- A/B testing different versions of a page.
- Directing users to location-specific content based on their IP address.
Unlike a 301 redirect, a 302 redirect does not transfer SEO value to the new URL, as search engines treat it as a temporary change.
How to Implement a Redirect in PHP
The most common method for implementing a redirect in PHP is by using the header()
function. Below are step-by-step instructions and examples for creating redirects.
1. Basic 301 Redirect
To create a permanent redirect, use the following code:
header("HTTP/1.1 301 Moved Permanently");
header("Location: https://example.com/new-url");
exit();
?>
Alternatively, you can use a shorthand method:
header("Location: https://example.com/new-url", true, 301);
exit();
?>
The exit()
function ensures that no further code is executed after the redirect, preventing unexpected behavior.
2. Relative URL Redirect
If you need to redirect users to a relative URL within the same domain, you can use:
header("Location: /new-page");
exit();
?>
This is useful for internal page redirects without specifying the full URL.
3. Conditional Redirects
For dynamic redirects based on user input or conditions, use PHP logic:
if ($_SERVER['HTTP_USER_AGENT'] == 'Mobile') {
header("Location: https://example.com/mobile-version");
} else {
header("Location: https://example.com/desktop-version");
}
exit();
?>
Always validate and sanitize user input to prevent security vulnerabilities such as open redirects.
Best Practices for PHP Redirects
To ensure that your redirects are effective and secure, follow these best practices:
1. Set the Correct HTTP Status Code
Always specify the appropriate status code (301 or 302) when creating a redirect. If omitted, PHP defaults to a 302 redirect, which may not be suitable for permanent changes.
2. Place Redirects Before Output
The header()
function must be called before any output (e.g., HTML or echo statements) is sent to the browser. Otherwise, a "headers already sent" error will occur.
3. Use the exit()
Function
Terminate the script immediately after sending the redirect header to prevent further code execution and potential errors.
4. Avoid Redirect Loops
Ensure that your redirect logic does not create infinite loops, which can lead to errors and degrade website performance.
5. Validate User Input
When using dynamic redirects, validate and sanitize all user input to prevent open redirect vulnerabilities. Malicious actors could exploit unvalidated input to redirect users to harmful websites.
6. Test Redirects Thoroughly
Always test your redirects to ensure they work as expected. Use tools like browser developer tools or online redirect checkers to verify the status code and destination URL.
Common Use Cases for PHP Redirects
1. SEO and Website Restructuring
When restructuring a website or migrating to a new domain, use 301 redirects to maintain SEO rankings and ensure a seamless user experience.
2. Device-Based Redirects
Redirect users to mobile-optimized or desktop versions of your website based on their device type. This improves user experience and engagement.
3. Language or Location-Specific Redirects
Direct users to language-specific or region-specific pages based on their IP address or browser settings. Use 302 redirects for temporary changes and 301 redirects for permanent ones.
Security Considerations
While PHP redirects are powerful, they can introduce security risks if not implemented correctly. Follow these guidelines to mitigate vulnerabilities:
- Prevent Open Redirects: Validate and sanitize all input used in dynamic redirects.
- Keep Software Updated: Ensure your server and PHP environment are up-to-date with security patches.
- Monitor Redirects: Regularly review your redirect logic to identify and fix potential issues.
Conclusion
Redirects are an indispensable tool in web development, enabling developers to manage user navigation, preserve SEO rankings, and enhance user experience. By understanding the types of redirects, implementing them correctly using PHP's header()
function, and adhering to best practices, you can ensure seamless and secure redirection on your website. Always test your redirects thoroughly and stay vigilant against potential security risks to maintain an optimal browsing experience for your users.